Longest Palindromic Substring
Get started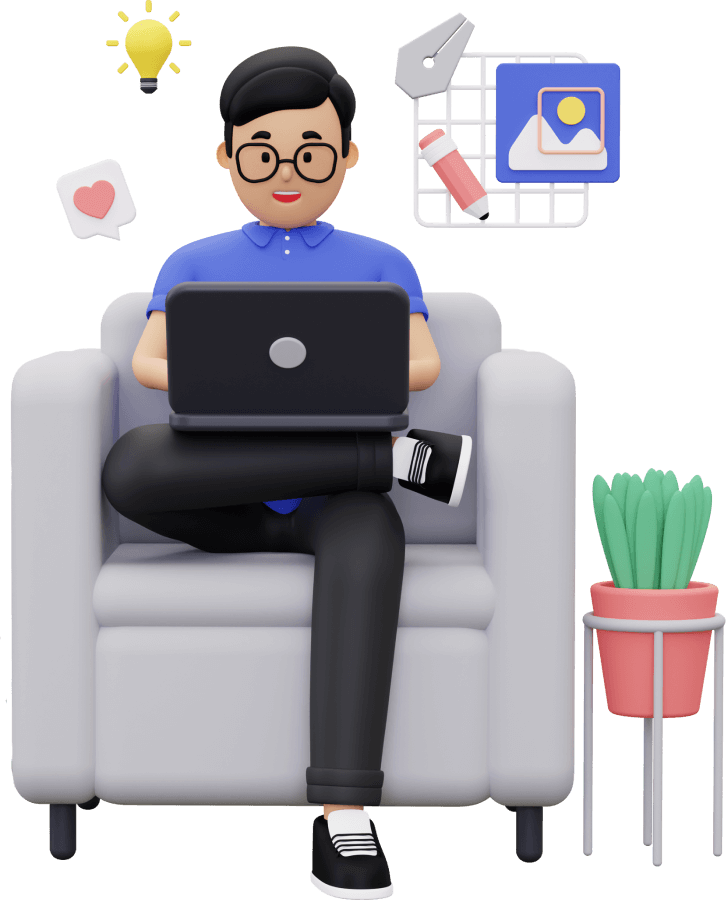
জয় শ্রী রাম
🕉Problem Statement:
Given a string s, return the longest palindromic substring in s.Example 1:
Input: s = "babad"
Output: "bab"
Note: "aba" is also a valid answer.
Example 2:
Input: s = "cbbd"
Output: "bb"
Example 3:
Input: s = "a"
Output: "a"
Example 4:
Input: s = "ac"
Output: "a"
Solution:
Please go through Dynamic Programming for Problems with 1 String and Palindrome first and try to solve this problem before looking at the code below. I am not adding any explanation here since after going through the aforementioned chapters everything will start making sense in the code below.Java Code:
Login to Access Content
Python Code:
Login to Access Content
Space Optimization:
The time complexity and space complexity of above algorithm are both O(n2) where n = length of the given string. We can optimize the space and can solve the problem in constant time.
This is not a Dynamic Programming approach. Instead of keeping the dp[][] array what we will do is: we will consider each and every index in the given string and we will check how long of a palindrome we get treating that index as the center of a palindrome. Since in a palindrome with even number of characters there are two center characters (example: in "abccba" there are two center characters "cc") and in a palindrome with odd number of characters there are only one center character (for example: in "abcba" there is only one center character which is 'c'), for every index we would compute palindromes with both odd length and even length. At the end we return palindrome with the overall maximum length.
Java Code:
Login to Access Content
Python Code:
Login to Access Content